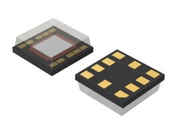
BH1792 I2C Heart rate Sensor
BH1792GLC is optical sensor for heart rate monitor IC in which LED driver, green light and IR detection photodiode are incorporated. This device drives LED and provides the intensity of light reflected from body.
In Espruino it is supported with the BH1792 (About Modules) module.
Wiring
If wiring the bare sensor up, consult the datasheet. For the breakout board connect GND, VCC (3.3v) and SDA/SCL/INT pins to GPIO on Espruino.
Software
Take green sensor readings at 32Hz, streaming:
var i2c = new I2C();
i2c.setup({sda:YOUR_SDA_PIN, scl:P.YOUR_SCL_PIN});
var h = require("BH1792").connect(i2c, {
irq : YOUR_INT_PIN,
callback : function(d) {
// 'd' is a Uint16Array of green light values at 32Hz (by default)
print(d.toString());
}});
// start
// the result of h.getDefaultOptions can be modified to allow different
// speeds or meauring modes - see the sourse
h.start(h.getDefaultOptions());
// call h.stop() to stop.
Or to take single readings with the IR LED (for example to see if it's being worn):
var i2c = new I2C();
i2c.setup({sda:YOUR_SDA_PIN, scl:P.YOUR_SCL_PIN});
var h = require("BH1792").connect(i2c, {
irq : YOUR_INT_PIN,
callback : function(d) {
// 'd' is an object of the form: { "irOff": ..., "irOn": ..., "greenOff": ..., "greenOn": ... }
print(d);
}});
// take a new reading every second, IR only
setInterval(function() {
h.start(h.getSingleOptions(true));
},1000);
Module reference
// Get a default set of options for streaming green HR readings that can be modified
BH1792.prototype.getDefaultOptions = function () { ... }
// Get a default set of options for taking a single reading - either green (ir=false) or IR (ir=true)
BH1792.prototype.getSingleOptions = function (ir) { ... }
// Start measurements
BH1792.prototype.start = function (o) { ... }
// Stop measurements
BH1792.prototype.stop = function () { ... }
/* Reads from the FIFO for streaming operation, return only data for green with light on.
Returns data as a Uint16Array of LED on readings
This is used internally
*/
BH1792.prototype.getFIFO = function () { ... }
/* Reads the current measurement data for non-streaming operation, also clears IRQ
Returns data in the form { "irOff": ..., "irOn": ..., "greenOff": ..., "greenOn": ... }
This is used internally
*/
BH1792.prototype.getMeasurements = function () { ... }
/* Connect to BH1792 and return an instance of BH1792.
options = {
addr, // I2C address (optional)
irq, // irq pin (optional)
callback // data callback - see getFIFO(if streaming)/getMeasurements(single) for data format
}
*/
exports.connect = function (i2c, options) { ... }
Using
(No tutorials are available yet)
This page is auto-generated from GitHub. If you see any mistakes or have suggestions, please let us know.